6 Design and Development of an Autonomous Sumo Robot
Vishwas Bedekar
1) Sumo Robot
A sumo robot is an autonomous robot that is fully programmed to function with predetermined tasks. As the sumo wrestlers fight inside the ring and try to defeat the opponent by bringing them down to the floor; a sumo robot must locate the opponent in a ring and try to push it out of the ring to win. The typical shape of the arena/ring are circular or square. Sumo robots are some of the most sophisticated machines designed and engineered for optimum performance. Figure 1 shows several designs of sumo robots used in competitions.
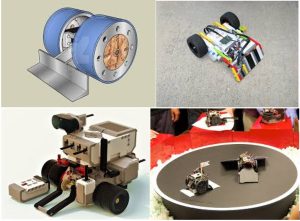
There are several weight categories that are available for competitions such as nano, micro, mini, mega and heavyweight. Each category has the size and weight criteria that must be met by its designers. The size varies between 1 inch X 1 inch X 1 inch to 24 inch X 24 inch X 24 inch. Other design considerations include materials used for components, electronic and electrical components, defense components, programming language and strategy, sensors and actuators and the rules of the competition. The following sections will discuss each of these design parameters in detail.
2) Physical parameters – size and weight
The size of the sumo robot is predetermined by the competition’s manuals. Most common sizes start at 1 inch by 1 inch by 1 inch for the “nano” bot size, 2 inch by 2 inch by 2 inch for “micro” bot, 4 inch by 4 inch by 4 inch for “mini” sumo bot, 8 inch by 8 inch by 8 inch for “mega” bot and 24 inch by 24 inch by 24 inch for a “heavyweight” sumo robot. Some competitions may define sizes other than ones mentioned above such as National Robotics Challenge defines 20 inch by 20 inch by 20 inch for sumo robots at various levels – middle school, high school, and post-secondary. The weight categories vary from 1 pound for nano sumo robot up to 50 pounds for heavyweight sumo robots.
3) Materials and mechanical parameters
Most common materials used in the build of sumo robots are polymers and metal alloys. Some competitions such as VEX robotics competitions specify certain materials/kits that participants can use to build their robots. Steel and aluminum alloys are used when the robot needs to “gain” weight. Polymers such as PTFE, HDPE, TPE, PLA, Acetal, ABS plastic etc. can be used to design the body of the robot. Key required properties are high impact resistance, good machinability, toughness, wear resistance, medium to high tensile strength, high density, good strength to weight ratio, high durability and endurance strength. Table 1 below shows properties of most common materials used in the design of sumo robots.
Table 1: Most common materials used in the design of sumo robots
Properties Steel Aluminum ABS plastic HDPE PLA TPE
Density (g/cm3) 7.85 2.7 1.05 0.941 – 0.965 1.24 0.99
Tensile strength (MPa) 276 – 1882 90 22 21 – 42 50 15
Melting point (°C) 1370 – 1510 660 190 – 250 120 – 190 120 – 170 230
Hardness (BHN) 86 – 388 15 46 – 100 180 – 200 254 83
Electrical resistivity (Ωm) 10-7 10-8 1015 1016 – 1017 108 – 1015 1012 – 1016
Thermal conductivity (W/m.K) 24.3 – 65.2 237 0.15 – 0.2 0.4 – 0.5 0.13 0.159
4) Programming and Control
The sumo robot is programmed for three objectives i.e. maneuvering or moving inside the ring, detection of opponent sumo robot, and detection of the edge of the ring. Once, the opponent is detected, sumo will execute the “push” programming steps to take the opponent out of the ring. Arduino IDE is the most common programing language used in autonomous sumo robots. Other languages used include Python, C++ and Scratch or Blockly (graphical programming). Controlling motors to execute command such as move forward, move backward, turn left or right, thrust forward and stop. Depending on the number of sensors used in the robot assembly, each sensor is integrated into the program code so that an appropriate action is taken based on its feedback. Example program is shown below:
Example Program
// Motor Pins
#define LEFT_MOTOR_FORWARD 9
#define LEFT_MOTOR_BACKWARD 10
#define RIGHT_MOTOR_FORWARD 11
#define RIGHT_MOTOR_BACKWARD 12
// Sensor Pins
#define EDGE_SENSOR_LEFT A0
#define EDGE_SENSOR_RIGHT A1
#define PROX_SENSOR_FRONT A2
// Thresholds
#define EDGE_THRESHOLD 500 // Adjust based on edge sensor calibration
#define PROX_THRESHOLD 300 // Adjust based on opponent distance
void setup() {
// Set motor pins as outputs
pinMode(LEFT_MOTOR_FORWARD, OUTPUT);
pinMode(LEFT_MOTOR_BACKWARD, OUTPUT);
pinMode(RIGHT_MOTOR_FORWARD, OUTPUT);
pinMode(RIGHT_MOTOR_BACKWARD, OUTPUT);
// Set sensor pins as inputs
pinMode(EDGE_SENSOR_LEFT, INPUT);
pinMode(EDGE_SENSOR_RIGHT, INPUT);
pinMode(PROX_SENSOR_FRONT, INPUT);
Serial.begin(9600); // For debugging
}
void moveForward() {
digitalWrite(LEFT_MOTOR_FORWARD, HIGH);
digitalWrite(LEFT_MOTOR_BACKWARD, LOW);
digitalWrite(RIGHT_MOTOR_FORWARD, HIGH);
digitalWrite(RIGHT_MOTOR_BACKWARD, LOW);
}
void moveBackward() {
digitalWrite(LEFT_MOTOR_FORWARD, LOW);
digitalWrite(LEFT_MOTOR_BACKWARD, HIGH);
digitalWrite(RIGHT_MOTOR_FORWARD, LOW);
digitalWrite(RIGHT_MOTOR_BACKWARD, HIGH);
}
void turnLeft() {
digitalWrite(LEFT_MOTOR_FORWARD, LOW);
digitalWrite(LEFT_MOTOR_BACKWARD, HIGH);
digitalWrite(RIGHT_MOTOR_FORWARD, HIGH);
digitalWrite(RIGHT_MOTOR_BACKWARD, LOW);
}
void turnRight() {
digitalWrite(LEFT_MOTOR_FORWARD, HIGH);
digitalWrite(LEFT_MOTOR_BACKWARD, LOW);
digitalWrite(RIGHT_MOTOR_FORWARD, LOW);
digitalWrite(RIGHT_MOTOR_BACKWARD, HIGH);
}
void stopMotors() {
digitalWrite(LEFT_MOTOR_FORWARD, LOW);
digitalWrite(LEFT_MOTOR_BACKWARD, LOW);
digitalWrite(RIGHT_MOTOR_FORWARD, LOW);
digitalWrite(RIGHT_MOTOR_BACKWARD, LOW);
}
void loop() {
// Read sensor values
int edgeLeft = analogRead(EDGE_SENSOR_LEFT);
int edgeRight = analogRead(EDGE_SENSOR_RIGHT);
int proxFront = analogRead(PROX_SENSOR_FRONT);
// Debugging: Print sensor values
Serial.print(“Edge Left: “);
Serial.print(edgeLeft);
Serial.print(” Edge Right: “);
Serial.print(edgeRight);
Serial.print(” Proximity: “);
Serial.println(proxFront);
// Edge detection: Avoid falling out of the ring
if (edgeLeft > EDGE_THRESHOLD) {
moveBackward();
delay(500); // Reverse for a moment
turnRight(); // Turn away from edge
delay(500);
return;
}
if (edgeRight > EDGE_THRESHOLD) {
moveBackward();
delay(500); // Reverse for a moment
turnLeft(); // Turn away from edge
delay(500);
return;
}
// Proximity detection: Charge toward opponent
if (proxFront > PROX_THRESHOLD) {
moveForward();
} else {
// Search for the opponent by turning
turnLeft();
}
}
5) Motors and drivers
The primary purpose of motors is to move the robot around the arena and allow maximum traction in the wheels so that it is difficult for the opponent robot to push the sumo out. A high torque motor/servo motor/DC motor is preferable compared to a stepper motor. The motor controller/driver will be chosen based on the class and specifications of the motor. For example, a uxcell DC 24V 80RPM Worm Gear Motor 10kg-cm Reversible High Torque Speed Reduce Turbine Electric Gearbox Motor 8mm Shaft can be coupled with a Cytron 10A 5-30V Dual Channel DC Motor Driver. The Cytron driver has several advantages: it provides bidirectional control for two brushed DC motors, high voltage range 5V – 30V DC, high current up to 10 A continuous for each channel, elimination of wear and tear of mechanical relay due to solid state components, does not require heat sink, and provide regenerative braking.
6) Sensors and range selection
Several types of sensors can be used for various purposes. Proximity sensors, IR sensors can be used to detect the opponent sumo robot, line sensors can be used to detect the limits of the arena. Analog as well as digital sensors can be used together for detecting the objects in the arena. The range of detection will need to be tuned and calibrated. For example, a Pololu Digital Distance Sensor 15cm can be used to detect an object that is within 6 inches from the sensor, whereas a Sharp/Socle GP2Y0A02YK0F Analog Distance Sensor 20-150cm can be used to determine the proximity of the opponent and can drive the sumo robot towards it and push it out of the arena. The Arduino reads distance values by using its built-in ADC to transform the sensor’s analog voltage into a digital value ranging from 0 to 1023. This digital value can then be converted into a distance in centimeters using a specific formula or lookup table provided for the sensor. Some sumo robots may choose to use vision sensors, however, embedding them into the sumo and their protection in the arena may become problematic.
7) Defense and pushing mechanisms
Offense could be the best defense in sumo competitions – high traction wheels with high gear ratio DC motors could help “stand ground” when the opponent pushes the sumo robot. However, activating the motors to push forward as soon as opponent is detected could result in avoiding being dragged into reverse by a much heavier or stronger robot. The robot can move in a circular direction initially to detect the opponent. Other defense mechanisms include using paint similar to the arena floor that may confuse the opponent in detection. Batteries used in robot construction for driving it in the arena will determine how much push force can be generated. The minimum force required to push the opponent can be calculated as follows:
F_push=μ_k*W
Where,
Fpush is the force required to push the opponent,
µk = kinetic coefficient of friction; can be assumed as 0.2 for most smooth plywood surfaces.
W = weight of the opponent sumo robot
8) Competition rules and regulations
The following competition rules are adapted from the National Robotics Challenge 2025 Contest Manual. The most important rules have been displayed below:
- The robot must be powered by electrical batteries.
- Robot must be self controlled and must use sensors to detect lines and opponent.
- Sumo robot can not degrade the surface or it will be disqualified.
- Dimensions and weight requirements will be checked by the competition officials before and after bouts and all robots must adhere to these specifications throughout the competition.
- Robot must have a 5 second delay before beginning to move/detect.
- All robots must have a visible RED latching emergency stop button on top of the robot.
Four possible starting combinations for the robots are shown below:

When any part of the robot crosses the outer white line of the arena in a contact situation with the other robot, it loses the bout and the robot that stays inside is declared winer. The decision of the judges will be final and binding.
9) Reliability and repeatability of the robot
For reliability and repeatability, the robot must be tested and evaluated. It is a good practice to test the robot against a heavier object and check the limits of its functions. Once, the sensors have been properly calibrated, they can be tested for detection and accuracy. The accuracy of the sensors can be also tested by resting the robot on a block (so that it cannot actually move) and then observing the functioning of the program and sensors when they are activated with an object moving in front. The program should drive the motors forward in the direction of the object. The ranges of sensors can also be tested by moving an object in front of the robot and finding its detection limits. A gradually increasing weight can be pushed by the sumo to find its limits and can be recorded for repeatability.